Intro
Fortunately, you are not tied only to the development of plugins for Maya 3D using C++, which implies a good knowledge of programming, debugging, memory management, etc.
Using Python has some advantages over C++:
(Please correct me if I made any mistake)
Python Plugin
Ok, but the purpose of this post is to have a small guide to setup Maya to be able to create a python plugin ... let's start.
Python packages are located here:
{Base Maya Directory}\Python\lib\site-packages
The only step needed to setup the Maya is by creating an environment variable in the system:
Variable name: PYTHONPATH
Value: {Base Maya Directory}\Python\lib\site-packages
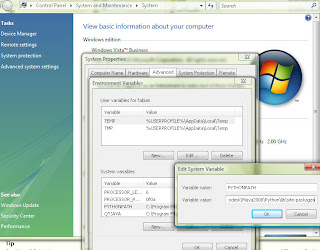
After this variable has been created, you should restart Maya in order to have it updated with the new variable pointing to the Python libraries.
Now, let's test if Python works. Try the following in the Maya Script Editor (Please make sure you are working in the Python Tab as shown in the following image
import maya.cmds as cmd
If you get the following error, then the Python variables were not set up properly. Please check your environment variable making sure that you have not included the "Maya" subfolder in the PYTHONPATH variable.
# Error: No module named maya.cmds
If no error is thrown, then you can start writing your Python scripts. Let's create a sphere with a radius of 3 units. The following command will create the new object named "Sphere1"
cmd.sphere(name='sphere1', radius=3)
Now you have a nice sphere, let's change some shape .... hmm something like an American Football ball (or whatever it's called ... pigskin?). To accomplish this, let's scale the sphere unevenly:
cmd.setAttr('sphere1.scale', 1, 1, 2, type='double3')
Note that the attribute is "scale" from the "sphere1" object. Also, we have a vector of 3 doubles with values 1, 1 and 2.
Finally, let's change our ball color. If you are thinking on something like setAttr sphere1.color, then you are wrong. Remember that color does not belong to the sphere itself but to the shader linked to it. By default, shader is lambert1, so let's change ball's color:
cmd.setAttr('lambert1.color', 0.63, 0.146, 0, type='double3')
Again, we modify "color" attribute from "lambert1" object, by updating color value using a 3-vector of doubles.
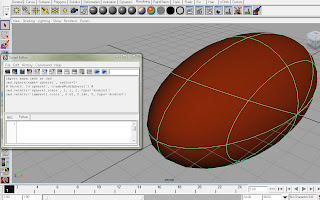
I hope this brief introduction will help you in your journey inside Maya scripting.
Fortunately, you are not tied only to the development of plugins for Maya 3D using C++, which implies a good knowledge of programming, debugging, memory management, etc.
Using Python has some advantages over C++:
- Multiplatform: Although C++ can run in multiple platforms, you have to fit the code to the machine and use different compilers and setting for each platform (although you use open source compilers). Anyway you have to recompile. There's no such in Python. It will work in every platform.
- Faster coding: Coding in Python is a way easier than C++. Few setup steps are required to develop applications in Python, and obviously, results will be seen faster.
Nothing is perfect, so these are the disadvantages I can think of:
- Speed: Being a scripted language, Python is a lot slower than C++ (Compiled)
- Low Level Programming: If you need to make son low level operations, then C++ (and assembler calls) is the right option.
(Please correct me if I made any mistake)
Python Plugin
Ok, but the purpose of this post is to have a small guide to setup Maya to be able to create a python plugin ... let's start.
Python packages are located here:
{Base Maya Directory}\Python\lib\site-packages
The only step needed to setup the Maya is by creating an environment variable in the system:
Variable name: PYTHONPATH
Value: {Base Maya Directory}\Python\lib\site-packages
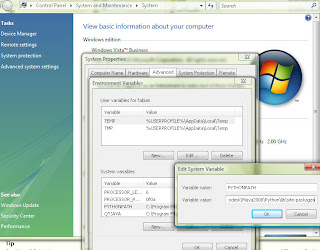
After this variable has been created, you should restart Maya in order to have it updated with the new variable pointing to the Python libraries.
Now, let's test if Python works. Try the following in the Maya Script Editor (Please make sure you are working in the Python Tab as shown in the following image
import maya.cmds as cmd
If you get the following error, then the Python variables were not set up properly. Please check your environment variable making sure that you have not included the "Maya" subfolder in the PYTHONPATH variable.
# Error: No module named maya.cmds
If no error is thrown, then you can start writing your Python scripts. Let's create a sphere with a radius of 3 units. The following command will create the new object named "Sphere1"
cmd.sphere(name='sphere1', radius=3)
Now you have a nice sphere, let's change some shape .... hmm something like an American Football ball (or whatever it's called ... pigskin?). To accomplish this, let's scale the sphere unevenly:
cmd.setAttr('sphere1.scale', 1, 1, 2, type='double3')
Note that the attribute is "scale" from the "sphere1" object. Also, we have a vector of 3 doubles with values 1, 1 and 2.
Finally, let's change our ball color. If you are thinking on something like setAttr sphere1.color, then you are wrong. Remember that color does not belong to the sphere itself but to the shader linked to it. By default, shader is lambert1, so let's change ball's color:
cmd.setAttr('lambert1.color', 0.63, 0.146, 0, type='double3')
Again, we modify "color" attribute from "lambert1" object, by updating color value using a 3-vector of doubles.
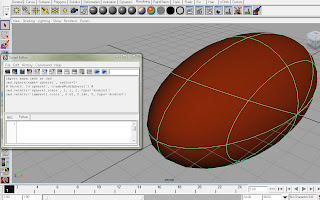
I hope this brief introduction will help you in your journey inside Maya scripting.
Comments
In C++ plugins, you don't have the same features level. It's more difficult to create a sphere in C++ than in Python of example. It's more difficult to create a simple window in C++ than in MEL. Some times, you are forced to use Maya API to call scrips commands from C++ because they just don't exist in C++ API. For example you can call 'MGlobal:executecommand("ployTriangulate;")' from C++.
But C++ is the only way to write efficient import/exporters, to access deep vertex information or mesh structure, and to have decent performances.